Universal links for Android and iOS

Quick guide to help you create your own short links
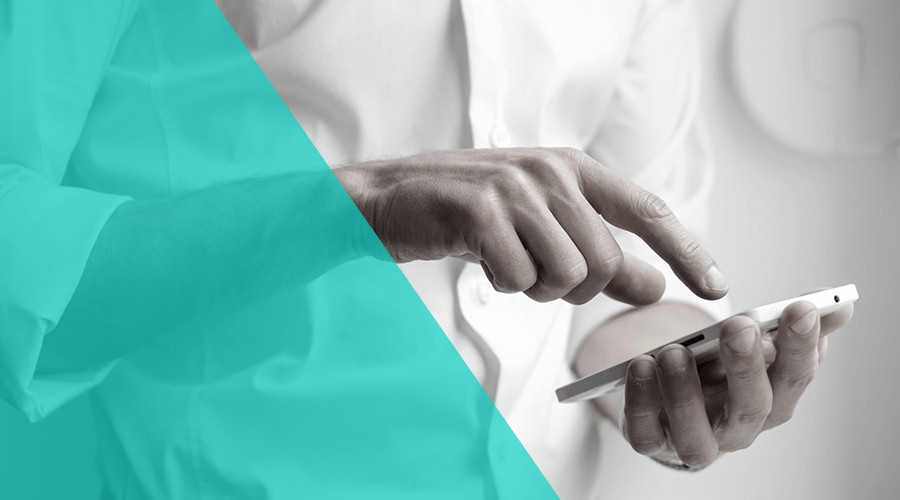
Last summer we've added support for Android App Links and iOS Universal Links. With this feature, you can have personalized short links that are able to intelligently take your users to the right place in your mobile apps when the user has your app installed, falling back to your app's App Store or Google Play page when they do not have it or eventually presenting an alternative web page when all other strategies fail.
Today, I would like to share with you how you can set this up for your own domain. Ideally, you will want to choose the shortest version of your current domain. Having a short domain name is key when you want to share these links through a medium like SMS or social networks, where space is at a premium.
For example if your current online presence is something like myshoponline.com, a short version of that domain would be something like mso.com. If you are lucky enough to still find a good short version of your current domain, that's a great starting point for implementing your own short links.
Setting up your web server
Great! You found the domain you want, you did your server setup and configured a valid HTTPS certificate. Please note that this certificate must be recognized by the operating system and custom root certificates are not supported. With that out of the way, you are now ready to start implementing universal links in your server.
iOS
In order to support universal links in iOS, you will need to upload an apple-app-site-association
file to your server. This is a JSON file that will be downloaded by an Apple device that has your app installed to determine what services the operating system will allow your app to use. Universal links is just one of the services that may be included in this file. This file should be located at:
https://yourdomain.com/.well-known/apple-app-site-association
In its basic form, this file will have the following contents:
{
"applinks": {
"details": [{
"appIDs": ["Application_Identifier_Prefix.Bundle_Identifier"],
"paths": ["/*"]
}]
}
}
You will need to provide at least one app identifier which consists of your app's identifier prefix followed by a dot and your app's bundle identifier.
There are several other definitions you can use to support internationalization, pattern matching, etc. In this post we will focus in the most basic form to get you quickly started. You can read more in Apple's own documentation.
You are now ready to start adding support for this domain in your own app. In your app, you will have to add the necessary entitlement (if not present yet) by adding a new capability. Go ahead and open the project’s Signing & Capabilities tab in Xcode and add the Associated Domains capability. Then add your domain by clicking the (+) icon in the bottom of the Domains table. Replace the placeholder domain by prepending the service you will use (applinks:) followed by your domain:

You are now ready to handle incoming universal links in your code. In your AppDelegate.swift
add the following:
func application(_ application: UIApplication,
continue userActivity: NSUserActivity,
restorationHandler: @escaping ([UIUserActivityRestoring]?) -> Void) -> Bool {
// userActivity.webpageURL will contain the link that triggered the app, handle it accordingly
return true
}
This is it. You can now handle incoming universal links as you see fit. Any user that has your app installed, will seamlessly be routed to your app when clicking any link from your domain.
Android
Android App Links are a special type of deep link that allow your website to immediately open the corresponding content in an Android app. Just like in iOS, you will configure your server so it can provide the relationship between your domain and an activity in your app. This will require that you add this JSON file at:
https://yourdomain.com/.well-known/assetlinks.json
In its basic form this file should contain the following:
[{
"relation": ["delegate_permission/common.handle_all_urls"],
"target": {
"namespace": "android_app",
"package_name": "APP_PACKAGE_NAME",
"sha256_cert_fingerprints": ["SHA256_FINGERPRINT"]
}
}]
You will need to provide the app's package name as declared in your app's build.gradle
file and one or more SHA256 fingerprints used to signed your app and all its versions (development or production).
You can use the following command to generate the fingerprint via the Java keytool:
keytool -list -v -keystore my-release-key.keystore
Pretty much like in iOS, you can add more definitions to this file. To learn more about Android App Links, please read Android's own documentation.
Now that you've configured your Digital Asset Links JSON file, you are ready to start handling these universal links in your app.
In your app's AndroidManifest.xml
, you will now declare an intent filter for the activity where you want to handle these links, as follows:
<activity ...>
<intent-filter android:autoVerify="true">
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data android:scheme="http" android:host="mydomain.com" />
<data android:scheme="https" />
</intent-filter>
</activity>
This will make sure the operating system is able to query your domain and verify all the hosts against the Digital Asset Links file you previously created. If successful, it will then establish that your app is the default handler for this specific URL pattern. This will remove the need for a disambiguation dialog usually presented in standard deep links.
Finally, you will now handle these links by implementing the following code, in the activity where you declared the intent filter:
public class YourActivity {
...
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
...
handleIntent(getIntent());
}
@Override
protected void onNewIntent(Intent intent) {
super.onNewIntent(intent);
handleIntent(intent);
}
protected void handleIntent(Intent intent) {
Uri universalLink = intent.getData();
//Handle the universalLink URI accordingly
}
...
}
This is all you need! You are now ready to handle universal links for both Android and iOS.
Cool, right?
We hope you can now also implement your own short links with support for universal links for your apps, but as mentioned in the beginning of this post, Notificare already offers a comprehensive solution for shorts URLs with support for redirects, landing pages and universal links for free in all our plans.
You're literally just one click away of seamlessly driving users from your website or SMS and email messages directly into the right content in your apps.
As always, if you have anything to add, doubts or just want to drop us a question, feel free to contact us via our Support Channel.