In a previous post, we demonstrated how to leverage Lottie to render beautiful Adobe AfterEffects animations in Android. This time around, we're turning to SwiftUI since its adoption has been increasing.
The problem
The Lottie library is available for iOS and macOS. However, it doesn't provide SwiftUI views yet.
We can work around this by creating our views, bridging the AnimationView
provided by the Lottie library into SwiftUI.
UIViewRepresentable
SwiftUI provides several well-built components out of the box. But there are times when that is not enough, and we need to fall back into UIKit for more flexibility or simply because what we need is not available in SwiftUI.
When facing such requirements, we can resort to UIViewRepresentable
. This allows us to bridge the gap between both worlds — UIKit & SwiftUI.
There are two key methods for conforming to UIViewRepresentable
.
makeUIView
— executed once, creating the underlying UIView.updateUIView
— executed several times as a side effect of updating the SwiftUI view.
Building a SwiftUI AnimationView
To convert the text above into concrete code, start by importing the Lottie module into your project.
Once that is done, create a LottieAnimationView
struct that conforms to the UIViewRepresentable
protocol.
Here's some code to better illustrate what needs to be done:
struct LottieAnimationView: UIViewRepresentable {
let animation: Lottie.Animation?
func makeUIView(context: Context) -> AnimationView {
AnimationView()
}
func updateUIView(_ view: AnimationView, context: Context) {
view.animation = animation
view.play()
}
}
This is it! 🎉
We have a fully working custom view that bridges Lottie's AnimationView
into the SwiftUI realm. But why stop here?
View modifiers
One final step into making a more robust view is adding methods that mutate other AnimationView
supported properties.
This is as simple as adding the properties to the custom view, the equivalent mutating methods and adjusting the updateUIView()
method to reflect the changes.
The following code serves as an example so you can add the necessary properties for your own use-case.
struct LottieAnimationView: UIViewRepresentable {
// more code ...
private var loopMode: Lottie.LottieLoopMode = .loop
func backgroundBehavior(_ behavior: Lottie.LottieBackgroundBehavior) -> Self {
var view = self
view.backgroundBehavior = behavior
return view
}
}
While one or two properties could be added to the struct's initializer, having several more would make it too complex. However, you can decide what to include in the initializer and what gets extracted into modifier methods.
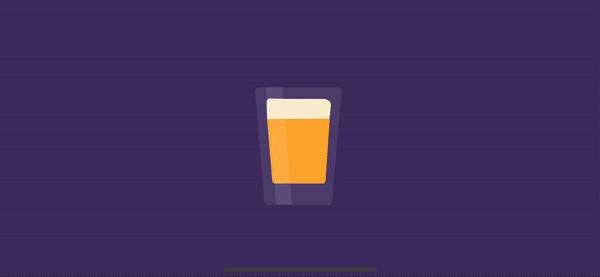
Conclusion
Should you want to take a look at the full code for the LottieAnimationView
, you can access this GitHub gist.
As always, we hope you liked this article and if you have anything to add, we are available via our Support Channel.