Intro to gRPC - What is gRPC?

An introduction to gRPC, the pro's and con's, and how to get started.
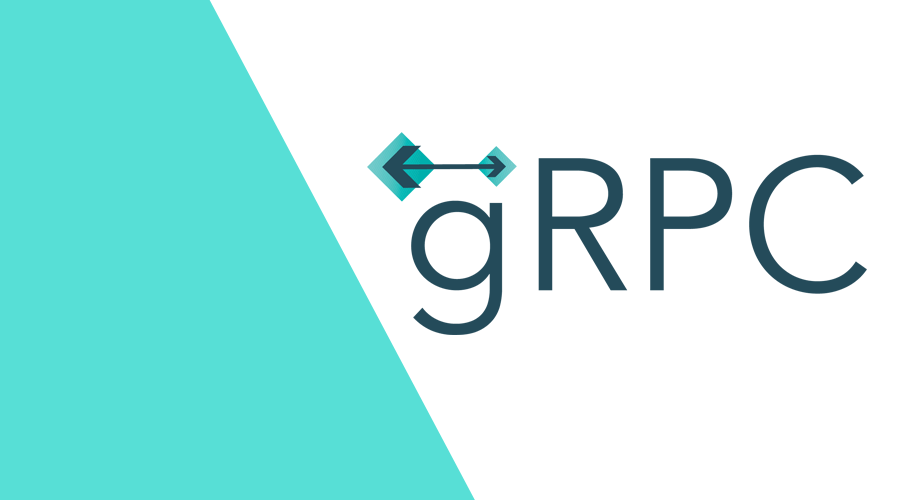
Creating and connecting services together using API's has been something I've done for most of my career as a Backend Developer. For most of that time I've been dealing with REST API's. While I have heard of gRPC, I never really took the time to look into it.
Recently, a friend of mine started working at a company that uses gRPC, and I was curious to see what it was all about. In this post, I'll explain what gRPC is, the pro's and con's, and how to get started.
What is gRPC?
To quote the official gRPC website:
gRPC is a modern open source high performance Remote Procedure Call (RPC) framework that can run in any environment. It can efficiently connect services in and across data centers with pluggable support for load balancing, tracing, health checking and authentication. It is also applicable in last mile of distributed computing to connect devices, mobile applications and browsers to backend services.
The concept of RPC is not new. It has been around for a long time, and there have been many implementations. The most popular one is probably SOAP, which is a standard for exchanging data between applications. gRPC is a modern alternative to SOAP, and it uses HTTP/2 as its transport protocol. This makes it very efficient, and it is also easy to use in modern microservice architectures.
To be honest, I have never actually used SOAP, so I can't really compare the two. My only experience is with REST, so my views will be based on that.
Let me start by saying that I realize that gRPC is not a replacement for REST. They are both very different, and they both have their own use cases. However, I think it is important to understand the differences between the two, so that you can make an informed decision on which one to use.
Protocol buffers (Protobuf)
The main ingredient of gRPC is Protocol Buffers (Protobuf). Protobuf is a language-neutral mechanism for serializing structured data. It is used to define the messages that are exchanged between the client and the server. The messages are defined using a simple interface description language (IDL). The IDL is used to generate data access classes in the desired language. The generated classes are then used by the client and server to send and receive messages.
A Protobuf message is defined using the following syntax:
message Person {
required string name = 1;
optional int32 id = 2;
optional string email = 3;
}
Besides the message definition, the IDL also contains service definitions. A service definition specifies the methods that can be called remotely with their parameters and return types. The following example shows a service definition:
message Person {
required string name = 1;
optional int32 id = 2;
optional string email = 3;
}
message SubscribeConfirmation {
required boolean success = 1;
optional string error = 2;
optional string message = 3;
}
service SubscriberService {
rpc SubscribeNewsletter (Person) returns (SubscribeConfirmation) {}
}
Code generation
I won't be going into the specifics of the code generation in this post, but you can find more information in the gRPC documentation.
The Protobuf compiler (protoc) is used to generate the data access classes from the IDL. The generated classes contain methods to serialize and deserialize the messages. The generated classes are language specific, and there are plugins available for most popular languages.
Once generated, the client and server can use the generated classes to send and receive messages. The client can call the remote methods by creating a request message, and passing it to the generated client stub. The server can implement the remote methods by creating a response message, and returning it from the generated server stub.
Pro's and Con's
Performance
Since gRPC is a binary protocol based on HTTP/2, it is a lot faster than REST, since it is a smaller payload and it is sent over a binary protocol. This is especially important for mobile and embedded applications, where bandwidth is limited and latency is important. The binary nature of gRPC makes it harder to debug, since you can't just open the request in your browser and see what is going on.
Code generation
Because gRPC uses Protobuf, it is possible to generate code for multiple languages. This makes it easy to use gRPC in a microservice architecture, where you might have services written in different languages. SDK's can be easily re-generated any time the Protobuf definition changes, so you don't have to worry about keeping the SDK's up to date. This also means that you have to generate the code for the language you are using. This can be a bit of a hassle, especially if you are using a language that is not supported by gRPC.
I do realize that Swagger is also a way to generate code for REST API's, but its output has been very hit-or-miss for me in the past. I have had to fix a lot of generated code, to the point it was better to just write the code myself. With gRPC I haven't had that problem yet, but I have only used it for a short time. Maybe I'll change my mind on this.
Conclusion
So far, gRPC seems like a very interesting technology. It is very fast, and it is easy to use. I'm looking forward to using it, and I am planning to write a follow-up post on my in-depth experience with it.
As always, we hope you liked this article and if you have anything to add, maybe you are suited for a Developer position in Notificare. We are currently looking for several positions, so please check out our careers page.